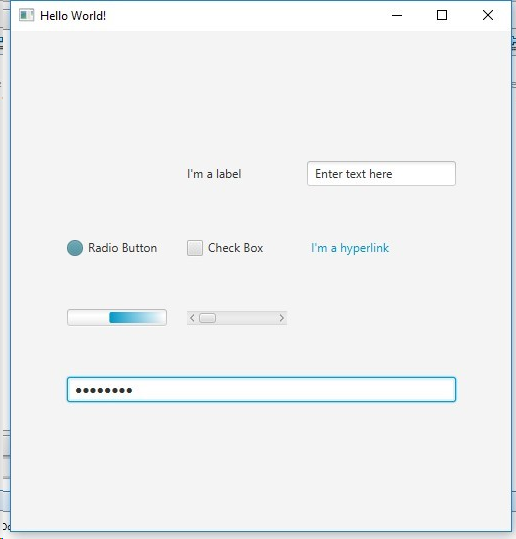
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package uicontrols;
import java.awt.Image;
import java.awt.Insets;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.CheckBox;
import javafx.scene.control.Hyperlink;
import javafx.scene.control.Label;
import javafx.scene.control.PasswordField;
import javafx.scene.control.ProgressBar;
import javafx.scene.control.RadioButton;
import javafx.scene.control.ScrollBar;
import javafx.scene.control.TextField;
import javafx.scene.image.ImageView;
import javafx.scene.layout.GridPane;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
/**
*
* @author mcold
*/
public class UIControls extends Application {
@Override
public void start(Stage primaryStage) {
Button btn = new Button();
btn.setText("I'm a button");
Label lbl = new Label("I'm a label");
TextField tf = new TextField("Enter text here");
Hyperlink hl = new Hyperlink("I'm a hyperlink");
RadioButton rb = new RadioButton("Radio Button");
rb.setStyle("-fx-base: #61a2b1");
CheckBox cb = new CheckBox("Check Box");
ProgressBar pb = new ProgressBar();
ScrollBar sb = new ScrollBar();
//Image imageHome = new Image("uicontrols/search.png");
//Button btn2 = new Button("Search", new ImageView(imageHome));
PasswordField pw = new PasswordField();
GridPane grid = new GridPane();
grid.setAlignment(Pos.CENTER);
grid.setHgap(20);
grid.setVgap(50);
Insets ins = new Insets(25,25,25,25);
//grid.setPadding(javafx.geometry.Insets.EMPTY);
//grid.setPadding(ins);
//grid.setPadding(new Insets(25,25,25,25));
//grid.setPadding(new Insets(25,25,25,25));
grid.add(btn, 0, 0);
grid.add(lbl, 1, 0);
grid.add(tf, 2, 0);
grid.add(rb, 0, 1);
grid.add(cb, 1, 1);
grid.add(hl, 2, 1);
grid.add(pb, 0, 2);
grid.add(sb, 1, 2);
grid.add(pw,0,3,3,1);
//grid.add(btn2, 2, 2);
StackPane root = new StackPane();
root.getChildren().add(btn);
Scene scene = new Scene(grid, 500, 500);
primaryStage.setTitle("Hello World!");
primaryStage.setScene(scene);
primaryStage.show();
}
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
launch(args);
}
}